https://docs.oracle.com/javase/tutorial/java/data/manipstrings.html
Creating Strings
String greeting = "Hello world!";
char[] helloArray = { 'h', 'e', 'l', 'l', 'o', '.' }; String helloString = new String(helloArray);
String Length
String palindrome = "Dot saw I was Tod"; int len = palindrome.length();
Concatenating Strings
string1.concat(string2);
"Hello," + " world" + "!
Note: The Java programming language does not permit literal strings to span lines in source files, so you must use the+
concatenation operator at the end of each line in a multi-line string. For example:String quote = "Now is the time for all good " + "men to come to the aid of their country.";
Creating Format Strings
Using
String's
static format()
method allows you to create a formatted string that you can reuse, as opposed to a one-time print statement. For exampleString fs; fs = String.format("The value of the float " + "variable is %f, while " + "the value of the " + "integer variable is %d, " + " and the string is %s", floatVar, intVar, stringVar); System.out.println(fs);
Converting Strings to Numbers
float a = (Float.valueOf("999")).floatValue();
Converting Numbers to Strings
String s = Double.toString(888.33);
Getting Characters and Substrings by Index
String anotherPalindrome = "Niagara. O roar again!"; char aChar = anotherPalindrome.charAt(9);

String anotherPalindrome = "Niagara. O roar again!"; String roar = anotherPalindrome.substring(11, 15);

Method | Description |
---|---|
String substring(int beginIndex, int endIndex) | Returns a new string that is a substring of this string. The substring begins at the specified beginIndex and extends to the character at index endIndex - 1 . |
String substring(int beginIndex) | Returns a new string that is a substring of this string. The integer argument specifies the index of the first character. Here, the returned substring extends to the end of the original string. |
Other Methods for Manipulating Strings
Here are several other
String
methods for manipulating strings:Method | Description |
---|---|
String[] split(String regex) String[] split(String regex, int limit) | Searches for a match as specified by the string argument (which contains a regular expression) and splits this string into an array of strings accordingly. The optional integer argument specifies the maximum size of the returned array. Regular expressions are covered in the lesson titled "Regular Expressions." |
CharSequence subSequence(int beginIndex, int endIndex) | Returns a new character sequence constructed from beginIndex index up until endIndex - 1. |
String trim() | Returns a copy of this string with leading and trailing white space removed. |
String toLowerCase() | Returns a copy of this string converted to lowercase or uppercase. If no conversions are necessary, these methods return the original string. |
Searching for Characters and Substrings in a String
Method | Description |
---|---|
int indexOf(int ch) | Returns the index of the first (last) occurrence of the specified character. |
int indexOf(int ch, int fromIndex) | Returns the index of the first (last) occurrence of the specified character, searching forward (backward) from the specified index. |
int indexOf(String str) | Returns the index of the first (last) occurrence of the specified substring. |
int indexOf(String str, int fromIndex) | Returns the index of the first (last) occurrence of the specified substring, searching forward (backward) from the specified index. |
boolean contains(CharSequence s) | Returns true if the string contains the specified character sequence. |
Note:
CharSequence
is an interface that is implemented by the String
class. Therefore, you can use a string as an argument for the contains()
method.Replacing Characters and Substrings into a String
Method | Description |
---|---|
String replace(char oldChar, char newChar) | Returns a new string resulting from replacing all occurrences of oldChar in this string with newChar. |
String replace(CharSequence target, CharSequence replacement) | Replaces each substring of this string that matches the literal target sequence with the specified literal replacement sequence. |
String replaceAll(String regex, String replacement) | Replaces each substring of this string that matches the given regular expression with the given replacement. |
String replaceFirst(String regex, String replacement) | Replaces the first substring of this string that matches the given regular expression with the given replacement. |
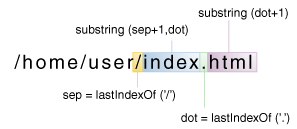
Comparing Strings and Portions of Strings
Method | Description |
---|---|
boolean endsWith(String suffix) | Returns true if this string ends with or begins with the substring specified as an argument to the method. |
boolean startsWith(String prefix, int offset) | Considers the string beginning at the index offset , and returns true if it begins with the substring specified as an argument. |
int compareTo(String anotherString) | Compares two strings lexicographically. Returns an integer indicating whether this string is greater than (result is > 0), equal to (result is = 0), or less than (result is < 0) the argument. |
int compareToIgnoreCase(String str) | Compares two strings lexicographically, ignoring differences in case. Returns an integer indicating whether this string is greater than (result is > 0), equal to (result is = 0), or less than (result is < 0) the argument. |
boolean equals(Object anObject) | Returns true if and only if the argument is a String object that represents the same sequence of characters as this object. |
boolean equalsIgnoreCase(String anotherString) | Returns true if and only if the argument is a String object that represents the same sequence of characters as this object, ignoring differences in case. |
boolean regionMatches(int toffset, String other, int ooffset, int len) | Tests whether the specified region of this string matches the specified region of the String argument.Region is of length len and begins at the index toffset for this string and ooffset for the other string. |
boolean regionMatches(boolean ignoreCase, int toffset, String other, int ooffset, int len) | Tests whether the specified region of this string matches the specified region of the String argument.Region is of length len and begins at the index toffset for this string and ooffset for the other string.The boolean argument indicates whether case should be ignored; if true, case is ignored when comparing characters. |
boolean matches(String regex) | Tests whether this string matches the specified regular expression. Regular expressions are discussed in the lesson titled "Regular Expressions." |
No comments:
Post a Comment